Speed Up Your Windows PC with These Tips
2024-09-05Understanding Python Syntax and Basic Commands
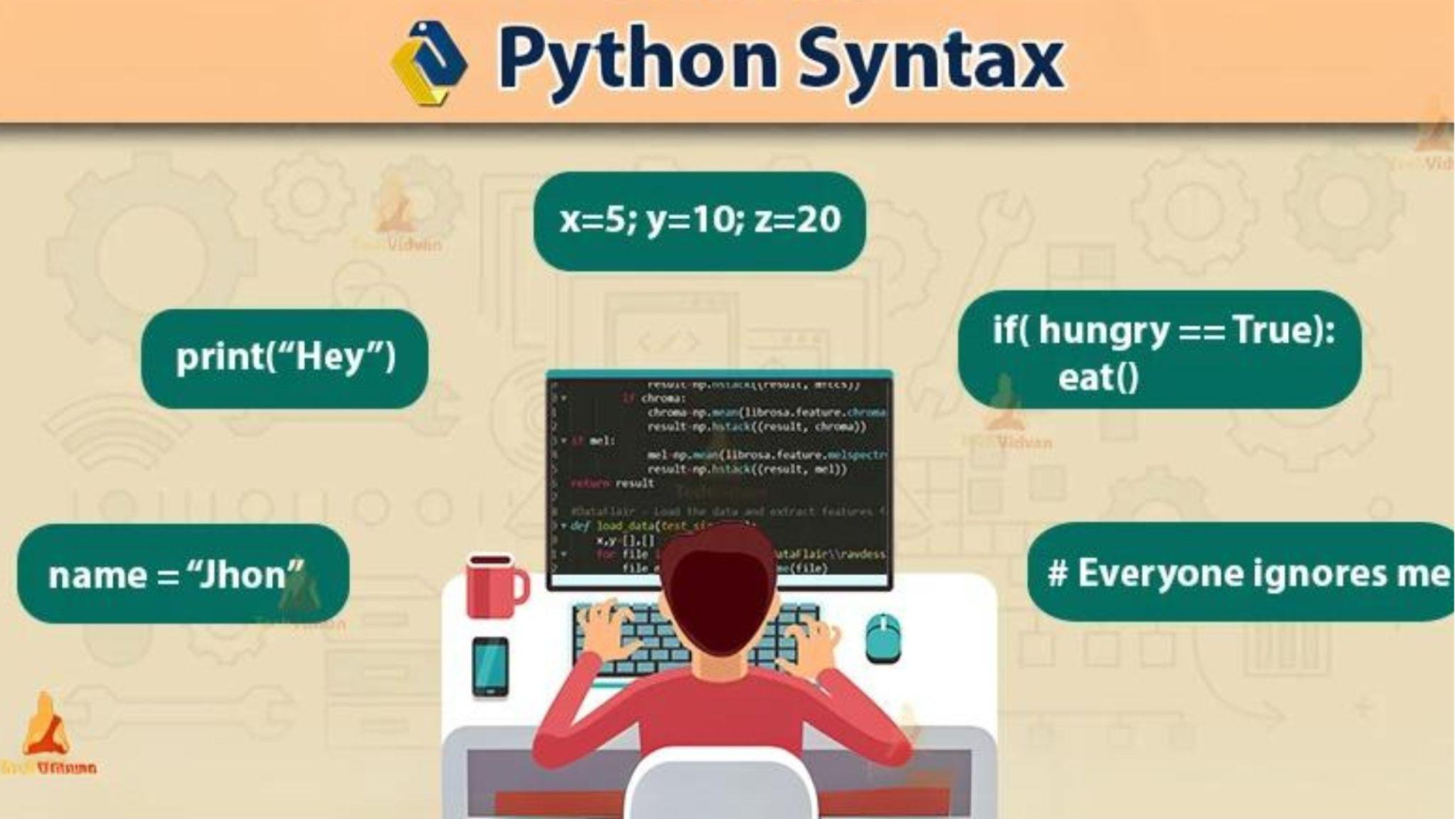
Python is like the Swiss Army knife of programming languages—simple, versatile, and incredibly powerful. If you’re just starting out in the world of coding, Python is a fantastic choice due to its readability and ease of use. In this guide, we’ll walk through the essentials of Python syntax and basic commands, giving you a solid foundation to start writing your own Python code. Whether you’re looking to learn Python basics or dive deeper into programming, this Python tutorial for beginners will set you on the right path.
What is Python Syntax?
At its core, Python syntax is about how you structure your code. Just like in any language, there are rules and conventions that must be followed to ensure that your code runs smoothly. Think of syntax as the grammar of programming—without it, your code would be like a sentence without punctuation, confusing and hard to understand.
Python’s syntax is known for being clean and easy to read. It emphasizes readability and reduces the complexity found in many other programming languages. This simplicity is achieved through its unique syntax rules:
Indentation: Instead of using curly braces or other symbols to define code blocks, Python uses indentation. Consistent indentation is crucial as it defines the structure of your code. For instance, all code inside a function, loop, or conditional statement must be indented equally.
def greet(name):
print(f”Hello, {name}!”)
Comments: Comments are notes in your code that help explain what’s going on. In Python, comments start with a #. They are ignored by the interpreter and are there purely for human readers.
# This prints a greeting
print(“Hello, world!”)
Basic Python Commands
Now that we’ve covered the basics of Python syntax, let’s explore some essential Python commands that you’ll use frequently. These commands will help you get started with writing functional Python programs.
1. Print Statement: The print() function is one of the first commands you’ll learn. It’s used to display output on the screen, which is invaluable for debugging and interacting with your code.
“`python
print(“Hello, world!”)
“`
2. Variables and Data Types: Variables are used to store data, and Python supports various data types like integers, floats, strings, and lists. You can assign values to variables using the = operator.
“`python
name = “Alice”
age = 30
height = 5.7
“`
3. Input Function: The input() function allows users to enter data. By default, it returns data as a string, so you may need to convert it to another type if necessary.
“`python
name = input(“Enter your name: “)
age = int(input(“Enter your age: “))
“`
4. Conditional Statements: Conditional statements let your program make decisions based on certain conditions. In Python, you use if, Elif, and else to control the flow of your program.
“`python
if age >= 18:
print(“You are an adult.”)
else:
print(“You are a minor.”)
“`
5. Loops: Loops allow you to execute a block of code repeatedly. Python has for and while loops. The for loop iterates over a sequence, while the while loop continues to execute as long as a condition is true.
“`python
# For loop
for i in range(5):
print(i)
# While loop
count = 0
while count < 5:
print(count)
count += 1
“`
6. Functions: Functions are reusable blocks of code that perform a specific task. They are defined using the def keyword and can accept parameters and return values.
“`python
def add_numbers(a, b):
return a + b
result = add_numbers(5, 3)
print(result)
“`
Basics of Python Programming
Getting comfortable with Python basics is key to becoming a proficient coder. Here’s a quick rundown of the essential topics you should focus on:
1. Data Structures: Learn about Python’s built-in data structures, such as lists, tuples, sets, and dictionaries. These structures help you organize and manipulate data efficiently.
2. File Handling: Python allows you to read from and write to files, which is crucial for handling external data. Familiarize yourself with file operations to manage data effectively.
3. Error Handling: Understanding how to handle errors with try and except blocks will help you manage exceptions and prevent your programs from crashing unexpectedly.
4. Modules and Packages: Learn how to organize your code into modules and packages. This practice enhances code management and reuse, making your programs more modular and maintainable.
Python Syntax Tutorial: Key Takeaways
1. Consistent Indentation: Ensure your code is consistently indented. Proper indentation defines code blocks and helps maintain readability.
2. Use Comments Wisely: Comments are your friend. They explain what your code does and help others (or your future self) understand it better.
3. Master Basic Commands: Get comfortable with basic Python commands like print(), variable assignments, and input functions. These commands form the foundation of your programming skills.
4. Practice Conditional Statements and Loops: Conditional statements and loops are powerful tools for creating dynamic and responsive programs. Practice using them to handle various scenarios in your code.
5. Explore Functions: Functions help organize and reuse code. Practice defining and using functions to make your code more efficient and easier to manage.
How to Learn Python Syntax
Here are some practical tips to help you learn Python syntax effectively:
- Explore Online Resources: Websites like Strategic Information Technology Services offer a wealth of tutorials and resources to help you get started with Python.
- Practice Regularly: The best way to learn Python is by doing. Work on small projects, solve coding challenges, and experiment with different syntax and commands.
- Join Coding Communities: Engage with online Python communities and forums. These platforms are great for asking questions, sharing knowledge, and collaborating with other learners.
- Read the Documentation: The official Python documentation is an excellent resource for understanding syntax rules and exploring Python’s extensive libraries.
Conclusion
Mastering Python syntax and basic commands is the first step towards becoming a skilled Python programmer. By understanding the code structure, practicing essential commands, and familiarizing yourself with Python’s rules, you’ll build a solid foundation with our services for more advanced programming concepts. Whether you’re looking to start a career in tech or simply exploring a new hobby, Python offers a user-friendly and versatile platform to bring your ideas to life.
For more resources and support on your Python journey, About Strategic Information Technology Services, where you can find additional tutorials and expert guidance to help you advance your coding skills.
Sign up for our twice-monthly newletter and be notified when a new blog post or event is happening!