Getting Started with Python: Installation and Setup
2024-08-30Writing Your First Python Program: Hello World!
2024-08-30Python Variables and Data Types Explained
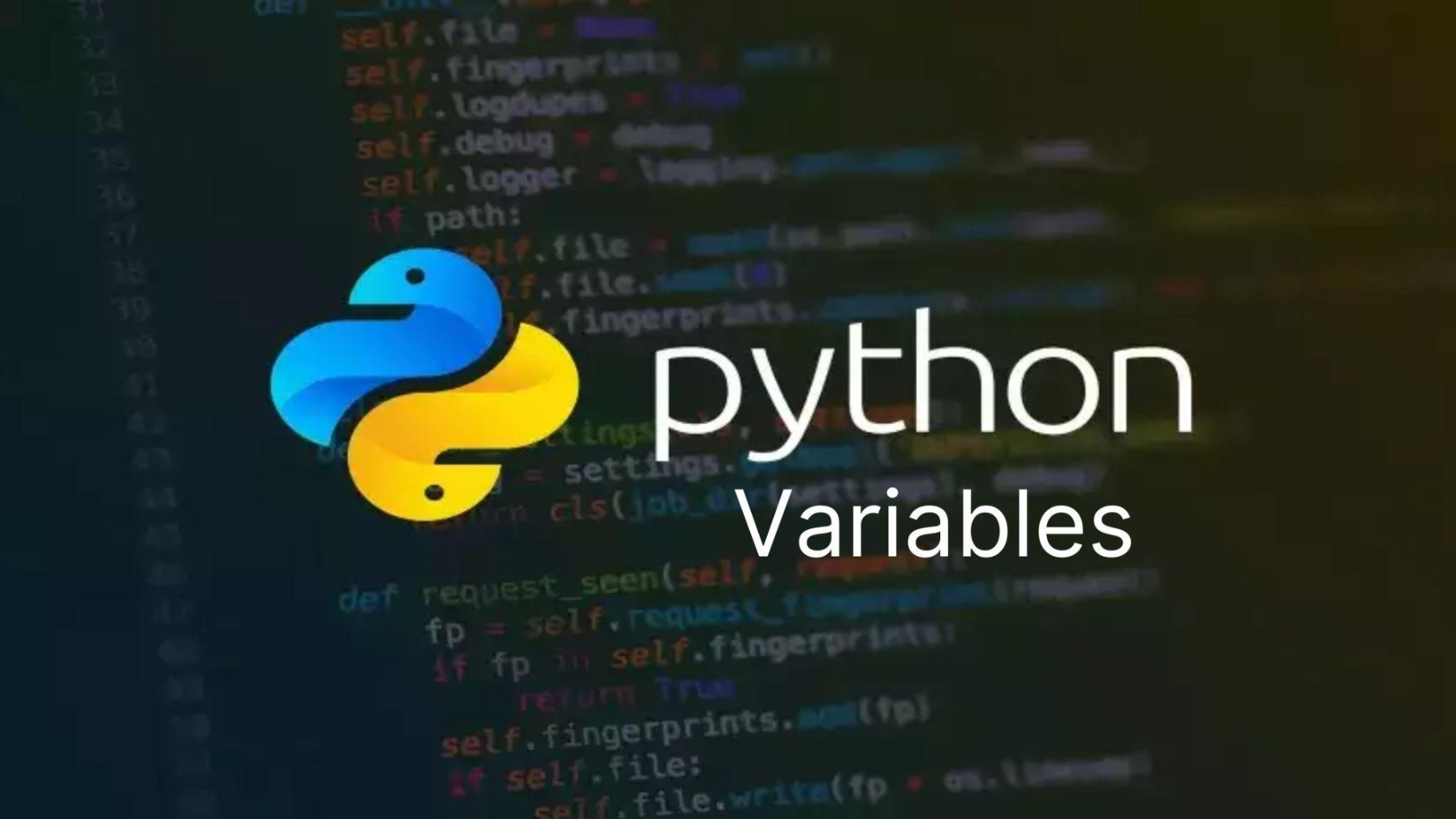
Python is one of today’s most popular programming languages, cherished for its straightforwardness and adaptability. One of the foundational concepts in Python is understanding variables and data types. In this blog, we’ll explore what Python variables are, the different data types available in Python, and how to use them effectively in your programs. By the end, you’ll have a solid understanding of Python variables and be ready to start coding your projects with the help of Strategic information technology services.
Understanding Python Variables
In Python, a variable is like a nickname that points to a value. You can think of a variable as a container that holds data that can be manipulated throughout your program. Assigning a value to a variable is simple and easy to do. Here’s a simple example:
x = 10
name = “Alice”
In this example, x is a variable that holds the integer value 10, and the name is a variable that holds the string value “Alice”.
Python Variable Naming Rules
When you name variables in Python, it’s important to follow specific rules and best practices.
- They cannot start with a number.
- Variable names can contain letters, numbers, and underscores. Variables cannot include spaces or special characters.
- Variable names are case-sensitive. In Python, “name” and “Name” are treated as distinct variables due to the language’s case-sensitive nature.
Here are examples of names that can and cannot be used as variables in programming.
Valid:
my_variable = 5
_name = “John”
number1 = 100
Invalid:
1st_number = 5 # Starts with a number
my-variable = “a” # Contains a special character
Data Types in Python
In Python, you don’t have to specify the type of a variable when you create it; the language figures out the type based on the value you assign. This flexibility makes Python programming more intuitive and less rigid compared to statically typed languages
Numeric Types in Python
Numeric data types are used to store numbers. Python supports several types of numeric data:
int Data Type
The `int` data type is used for storing whole numbers. For example:
age = 25
year = 2024
float Data Type
The float data type is used to store decimal numbers. For example:
pi = 3.14159
temperature = 98.6
Sequence Data Types in Python
Sequence data types are used to store collections of items. Python supports several sequence types:
str Data Type
The str data type is used to store strings, which are sequences of characters. For example:
greeting = “Hello, World!”
You can do many things with strings, like putting them together through concatenation:
first_name = “John”
last_name = “Doe”
full_name = first_name + ” ” + last_name
print(full_name) # Output: John Doe
list Data Type
The list data type is used to store an ordered collection of items, which can be of different types. You can change the content of lists because they are mutable. For example:
fruits = [“apple”, “banana”, “cherry”]
“Add ‘orange’ to the list of fruits.”
print(fruits) # Output: [“apple”, “banana”, “cherry”, “orange”]
tuple Data Type
The tuple data type is similar to a list, but it is immutable, meaning once it is created, you cannot change its content. For example:
coordinates = (10.0, 20.0)
print(coordinates) # Output: (10.0, 20.0)
Mapping Types in Python
Mapping data types are used to store collections of key-value pairs. Python supports the dict data type for mappings.
dict Data Type
The dict data type, also known as a dictionary, is used to store key-value pairs. For example:
person = {
“name”: “Alice”,
“age”: 30,
“city”: “New York”
}
print(person[“name”]) # Output: Alice
Other Common Data Types in Python
Python also supports several other data types, such as:
bool Data Type
The bool data type stores Boolean values, representing conditions as either True or False. For example:
is_active = True
is_admin = False
NoneType Data Type
The NoneType signifies that there is no value present. The only value of NoneType is None. For example:
data = None
Data Manipulation in Python
Python provides a rich set of operations for manipulating data. Here are a few common operations:
Arithmetic Operations
You can do math operations with numeric data types:
a = 10
b = 3
print(a + b) # Output: 13
print(a – b) # Output: 7
print(a * b) # Output: 30
print(a / b) # Output: 3.3333333333333335
String Manipulation
You can perform various operations on strings, such as slicing and formatting:
text = “Hello, World!”
print(text.upper()) # Output: HELLO, WORLD!
print(text[0:5]) # Output: Hello
print(f”{first_name} {last_name}”) # Output: John Doe
List Operations
You can perform various operations on lists, such as adding, removing, and sorting items:
numbers = [1, 3, 5, 2, 4]
numbers.sort()
Showing the numbers: [1, 2, 3, 4, 5]
numbers.remove(3)
print(numbers) # Output: [1, 2, 4, 5]
Dictionary Operations
You can perform various operations on dictionaries, such as adding and removing key-value pairs:
person[“age”] = 31
del person[“city”]
print(person) # Output: {‘name’: ‘Alice’, ‘age’: 31}
Basic Programs in Python
Import statements in Python are essential for adding extra functionality to your programs by allowing you to include and use code from external modules and libraries. These statements enable you to leverage pre-written code for various tasks, such as mathematical calculations, data manipulation, or web development, making your coding process more efficient. You can import entire modules, specific functions, or classes, and even use aliases to simplify long module names or avoid conflicts. Importing submodules is also possible, giving you access to specific components of a module. Overall, import statements enhance the versatility and power of your Python programs.
To solidify your understanding of Python variables and data types, let’s look at some basic programs.
Program 1: Calculate the Area of a Circle
This program computes the area of a circle based on its radius.
import math
radius = float {input(“Enter the radius of the circle “)}
area = math.pi * radius ** 2
print(f”The area of the circle is {area}”)
Program 2: Find the Maximum Number in a List
This program finds the maximum number in a list of numbers:
numbers = [10, 5, 20, 15, 25]
max_number = max(numbers)
print(f”The maximum number is {max_number}”)
Program 3: Reverse a String
This program reverses a given string:
text = input(“Enter a string: “)
reversed_text = text[::-1]
print(f”The reversed string is {reversed_text}”)
Conclusion
Understanding Python variables and data types is essential for writing efficient and effective Python programs. By mastering these basics, you can manipulate and store data in various ways, making your programs more versatile and powerful. Whether you’re a beginner or looking to brush up on your skills, knowing how to work with Python variables and data types is a fundamental step in your programming journey.
Using the right tools and libraries, like those offered in Python, you can build everything from simple scripts to complex applications. So, start experimenting with variables and data types in Python, and you’ll soon see the endless possibilities this versatile language has to offer. Happy coding!
Also Read,
Understanding Compliance and Data Sovereignty in Cloud Storage
Trends in IT Infrastructure and How to Stay Ahead
Sign up for our twice-monthly newletter and be notified when a new blog post or event is happening!